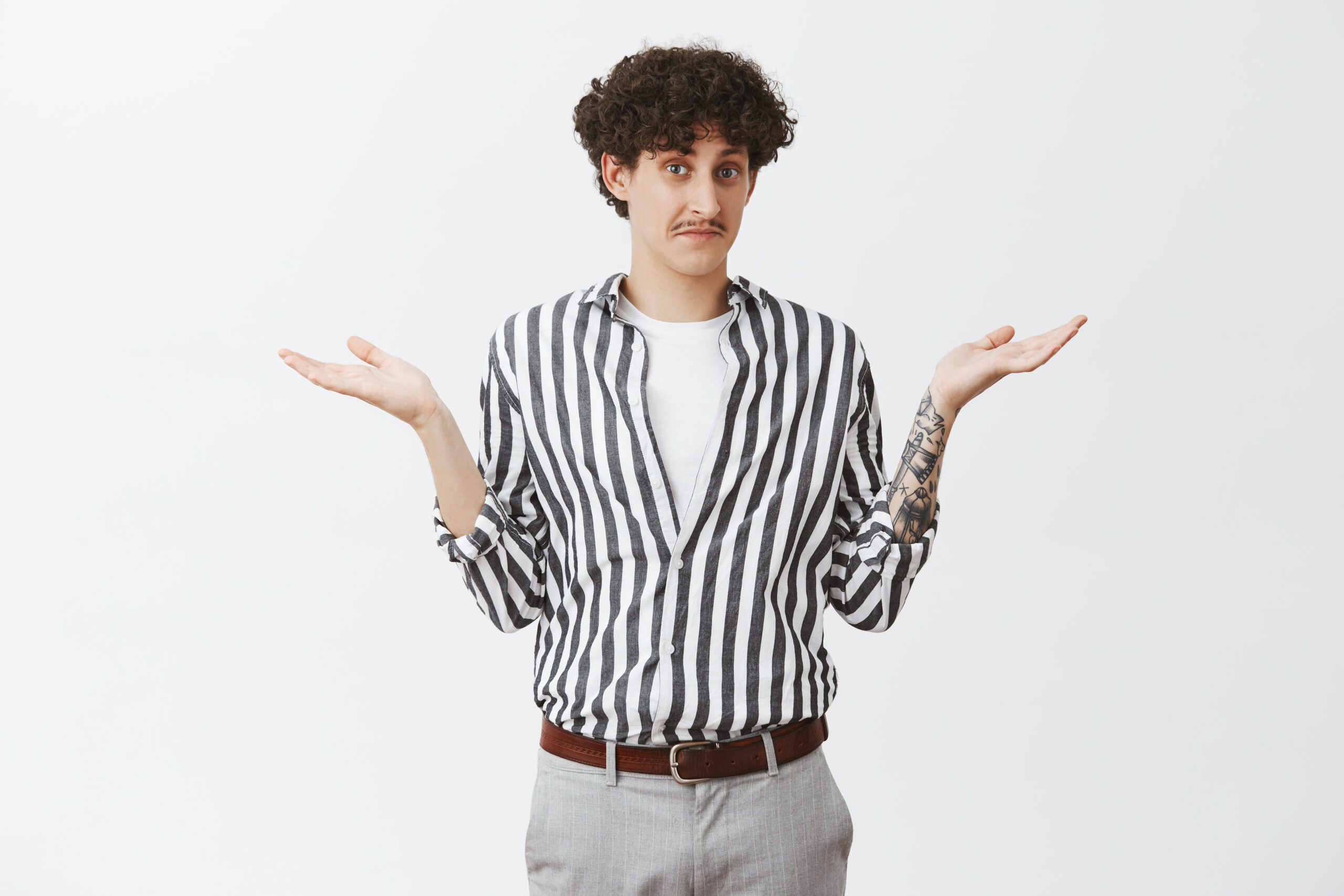
Learn How to Use Free Live Stock APIs: A Practical Guide
Ready to get your hands dirty with some real market data? If you’ve been following along, you already know what stock APIs are and why Insight Ease is awesome. Now let’s jump into the fun part – actually using them!
Getting Your API Access Ready
First things first – let’s get you set up with Insight Ease. Here’s your super simple checklist:
- Register for your free account
- Grab your API key
- Keep it somewhere safe (but don’t share it!)
Your First API Call (It’s Easy, Promise!)
Let me show you the simplest way to get started. Here’s a basic example using JavaScript:
// Your first stock API call!
const apiKey = 'your_api_key_here';
const symbol = 'AAPL'; // Let's check Apple's stock
fetch(`https://api.insightease.com/v1/stocks/${symbol}?apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Oops!', error));
What You’ll Get Back
You’ll get something cool like this:
{
"symbol": "AAPL",
"price": 178.45,
"change": 2.30,
"changePercent": 1.29,
"volume": 48291053
}
Cool Things You Can Build
Now that you’ve got the basics, here are some fun projects you can try:
1. Price Alert Dashboard
// Check if price crosses your target
function checkPriceAlert(symbol, targetPrice) {
fetch(`https://api.insightease.com/v1/stocks/${symbol}?apikey=${apiKey}`)
.then(response => response.json())
.then(data => {
if(data.price >= targetPrice) {
alert(`${symbol} hit your target price!`);
}
});
}
2. Portfolio Tracker
// Track multiple stocks at once
const portfolio = ['AAPL', 'GOOGL', 'MSFT'];
function updatePortfolio() {
portfolio.forEach(symbol => {
fetchStockData(symbol);
});
}
Pro Tips from My Experience
After playing with the API for a while, here are some cool tricks:
- Cache your data! Don’t call the API every second
“`javascript
const CACHE_DURATION = 60000; // 1 minute
let cachedData = {};
function getStockData(symbol) {
if (cachedData[symbol] && Date.now() – cachedData[symbol].timestamp < CACHE_DURATION) {
return cachedData[symbol].data;
}
// Fetch new data if cache expired
}
2. Handle errors gracefully
```javascript
function fetchStockData(symbol) {
fetch(`https://api.insightease.com/v1/stocks/${symbol}?apikey=${apiKey}`)
.then(response => {
if (!response.ok) throw new Error('Network error');
return response.json();
})
.catch(error => {
console.error(`Failed to fetch ${symbol}:`, error);
// Show user-friendly error message
});
}
Common Use Cases
Here’s what people are building with Insight Ease’s stock API:
For Websites
- Live stock tickers
- Market overview pages
- Portfolio tracking tools
For Trading Tools
- Price alert systems
- Trading signals
- Market analysis tools
Making it Look Pretty
Want to show off your data? Here’s a simple chart example using Chart.js:
function createStockChart(symbol) {
fetch(`https://api.insightease.com/v1/stocks/${symbol}/history?apikey=${apiKey}`)
.then(response => response.json())
.then(data => {
const ctx = document.getElementById('stockChart').getContext('2d');
new Chart(ctx, {
type: 'line',
data: {
labels: data.map(d => d.date),
datasets: [{
label: symbol,
data: data.map(d => d.price)
}]
}
});
});
}
Tips for Better Performance
- Batch your requests when possible
- Use WebSocket for real-time data
- Implement proper error handling
- Cache frequently accessed data
Troubleshooting Common Issues
Having problems? Here are quick fixes for common issues:
Rate Limits
// Add delay between requests
function delay(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function fetchWithDelay(symbol) {
await delay(1000); // 1 second delay
return fetch(`https://api.insightease.com/v1/stocks/${symbol}`);
}
Invalid API Key
Double-check your API key and make sure it’s being sent correctly in the request header.
Frequently Asked Questions
How often can I call the API?
With the free tier, you get plenty of calls – just don’t go crazy with it!
Can I get historical data too?
Yep! Just use the /history endpoint with your desired date range.
What about real-time updates?
Use WebSocket connections for real-time data – way more efficient than polling!
Is the free tier good enough for learning?
Absolutely! You get plenty of features to build cool stuff.
Ready to Level Up?
Now that you’ve got the basics down, try these challenges:
- Build a multi-stock dashboard
- Add price alerts
- Create a simple trading bot
- Make a portfolio analyzer
Remember, the best way to learn is by doing. Start small, test things out, and gradually add more features. And hey, if you build something cool, share it with the community!
Need more help? Check out the full API documentation. Happy coding!