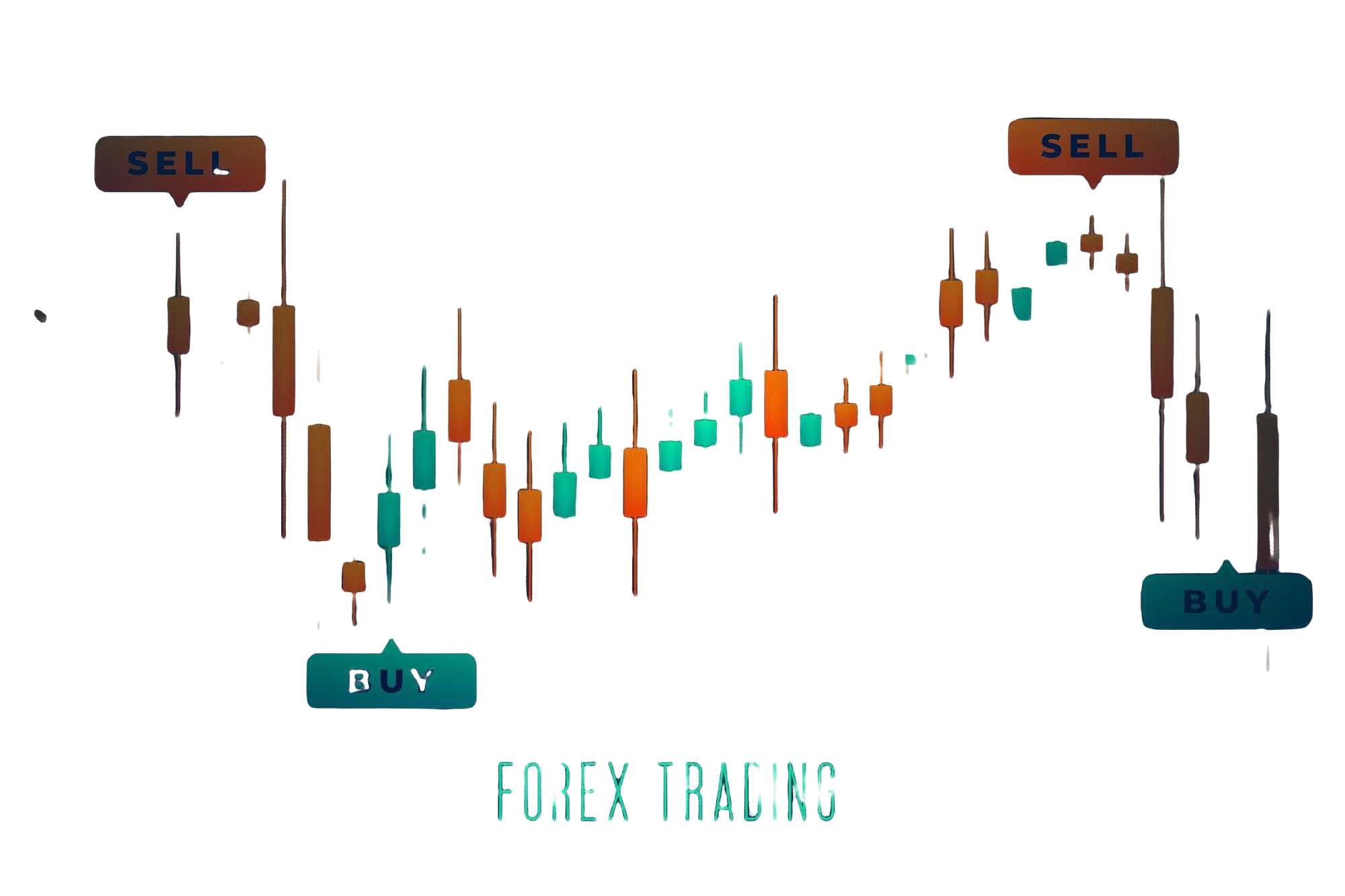
How to Handle Rate Limits with InsightEase Financial APIs
For developers building applications for brokers, investors, traders, and financial professionals, managing API rate limits is a critical skill. Exceeding these limits can disrupt data flow, impacting tools that rely on real-time financial data. Insightease provides robust APIs for forex, cryptocurrencies, stocks, and commodities, along with clear guidelines to handle rate limits effectively. This guide explains how to navigate these constraints and keep your app running smoothly.
Understanding Rate Limits in Financial APIs
Rate limits cap the number of API requests you can make within a specific timeframe, ensuring fair usage and system stability.
Why They Exist
Financial APIs, like those from InsightEase, deliver live and historical data to a wide audience. Limits prevent overload, maintaining performance for all users, from traders tracking markets to investors analyzing trends.
Impact on Your App
Exceeding limits can result in errors or temporary blocks, interrupting the experience for brokers and financial professionals who depend on timely updates.
Strategies to Manage Rate Limits
InsightEase (insightease.com) offers a developer-friendly approach to working within rate limits. Here’s how to handle them effectively.
Check Documentation
Start by reviewing InsightEase’s API documentation, available at insightease.com. It outlines specific limits (e.g., requests per minute) and provides usage tiers tailored to your project’s needs.
Implement Request Throttling
Space out your API calls to stay within bounds. Here’s a Python example to fetch stock data with a delay:
python
CollapseWrapCopy
import requests
import time
url = “https://api.insightease.com/v1/stocks/rates?symbol=AAPL”
headers = {“Authorization”: “Bearer YOUR_API_KEY”}
for _ in range(5):
response = requests.get(url, headers=headers)
if response.status_code == 200:
print(response.json()[‘rates’][‘AAPL’])
time.sleep(1) # Delay to respect rate limits
This snippet paces requests, avoiding limit violations.
Optimizing Data Usage
Reducing unnecessary calls preserves your quota and enhances app efficiency.
Cache Frequently Used Data
Store static or slow-changing data, like historical forex trends, locally. InsightEase’s real-time updates can then focus on live rates, balancing your request load.
Batch Requests
Combine multiple data points into a single call. For example:
python
CollapseWrapCopy
url = “https://api.insightease.com/v1/rates?symbols=EURUSD,BTCUSD,AAPL”
response = requests.get(url, headers=headers)
print(response.json()[‘rates’])
This retrieves forex, crypto, and stock data in one request, minimizing usage.
Handling Limit Exceedances
Even with careful planning, you might occasionally hit limits. Be prepared to manage these scenarios.
Monitor Response Headers
InsightEase includes rate limit details in response headers (e.g., remaining requests). Check these to adjust dynamically:
python
CollapseWrapCopy
response = requests.get(url, headers=headers)
remaining = response.headers.get(‘X-Rate-Limit-Remaining’)
print(f”Requests left: {remaining}“)
Graceful Error Handling
If limits are exceeded, handle errors smoothly:
javascript
CollapseWrapCopy
fetch(‘https://api.insightease.com/v1/crypto/rates?symbol=BTCUSD’, {
headers: { ‘Authorization’: ‘Bearer YOUR_API_KEY’ }
})
.then(response => {
if (response.status === 429) throw new Error(‘Rate limit exceeded’);
return response.json();
})
.catch(error => console.log(error.message));
This ensures your app remains user-friendly.
Conclusion
Handling rate limits with InsightEase financial APIs is straightforward when you understand the constraints and apply smart strategies. By throttling requests, optimizing data usage, and preparing for exceedances, developers can create reliable tools for brokers, investors, traders, and financial professionals. InsightEase (insightease.com) supports this process with real-time data, live charts, and seamless integration features like market analysis and an economic calendar. Visit insightease.com to explore their APIs and build your next financial application with confidence.