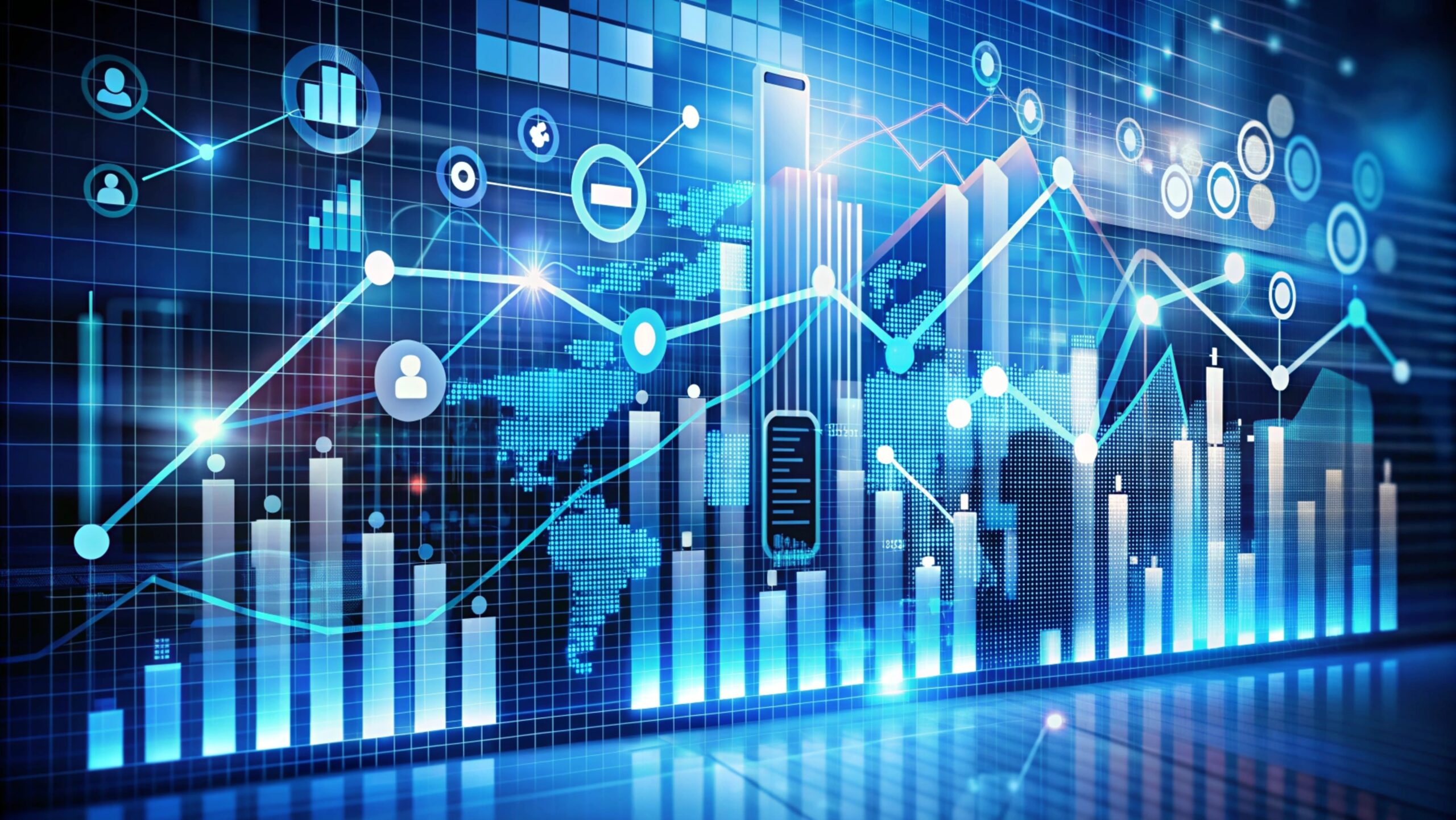
How Do I Implement Real-Time Updates in an API?
Introduction
In today’s fast-paced digital world, real-time data is crucial for financial markets, trading platforms, and web applications. APIs that provide live updates help ensure timely decision-making and efficient system performance. This article explores various methods for implementing real-time updates in an API, their benefits, and best practices.
Understanding Real-Time API Updates
Real-time API updates enable applications to receive instant data without requiring users to refresh the page manually. This is particularly important for industries like finance, where even a slight delay can impact trading decisions.
Methods for Implementing Real-Time Updates
1. WebSockets
WebSockets provide a full-duplex communication channel over a single TCP connection. Unlike traditional HTTP requests, WebSockets allow continuous data flow between the client and server.
Advantages:
- Persistent connection reduces latency
- Efficient use of bandwidth
- Ideal for financial applications and trading platforms
Implementation Example:
const socket = new WebSocket(“wss://api.insightease.com/realtime”);
socket.onopen = function() {
console.log(“Connection established”);
};
socket.onmessage = function(event) {
console.log(“New data received: “, event.data);
};
2. Server-Sent Events (SSE)
SSE is a one-way communication channel where the server pushes updates to the client over HTTP.
Advantages:
- Simpler to implement compared to WebSockets
- Efficient for applications that only require server-to-client updates
- Uses standard HTTP connections
Implementation Example:
const eventSource = new EventSource(“https://api.insightease.com/updates”);
eventSource.onmessage = function(event) {
console.log(“New data received: “, event.data);
};
3. Polling
Polling involves sending periodic requests to the server to check for updates.
Advantages:
- Simple to implement
- Works with any API
Disadvantages:
- Increased server load due to frequent requests
- Higher latency compared to WebSockets and SSE
Implementation Example:
setInterval(async () => {
const response = await fetch(“https://api.insightease.com/latest-data”);
const data = await response.json();
console.log(“New data received: “, data);
}, 5000); // Polling every 5 seconds
4. Long Polling
Long polling keeps the connection open until the server has new data, reducing the need for continuous requests.
Advantages:
- Reduces unnecessary server requests
- Works well when WebSockets are not an option
Implementation Example:
async function fetchUpdates() {
const response = await fetch(“https://api.insightease.com/updates”, { method: “GET” });
const data = await response.json();
console.log(“New data received: “, data);
fetchUpdates(); // Re-initiate the request after receiving data
}
fetchUpdates();
Choosing the Right Approach
The ideal method depends on the use case:
- For real-time financial data: WebSockets provide low-latency updates.
- For event-driven updates: SSE is efficient when only server-to-client communication is needed.
- For general use cases: Long polling balances efficiency and simplicity.
Best Practices for Real-Time API Implementation
- Optimize bandwidth usage: Send only necessary updates to reduce network load.
- Use authentication and encryption: Secure data transmission with API keys and HTTPS.
- Handle connection failures gracefully: Implement reconnection logic to maintain data flow.
- Monitor API performance: Use logging and analytics to detect issues early.
Conclusion
Real-time updates enhance the efficiency and responsiveness of applications, particularly in industries like finance and trading. By selecting the appropriate method—whether WebSockets, SSE, polling, or long polling—developers can ensure seamless data delivery. Insightease provides APIs that integrate real-time updates into financial platforms, offering reliable and accurate market data for traders and investors.