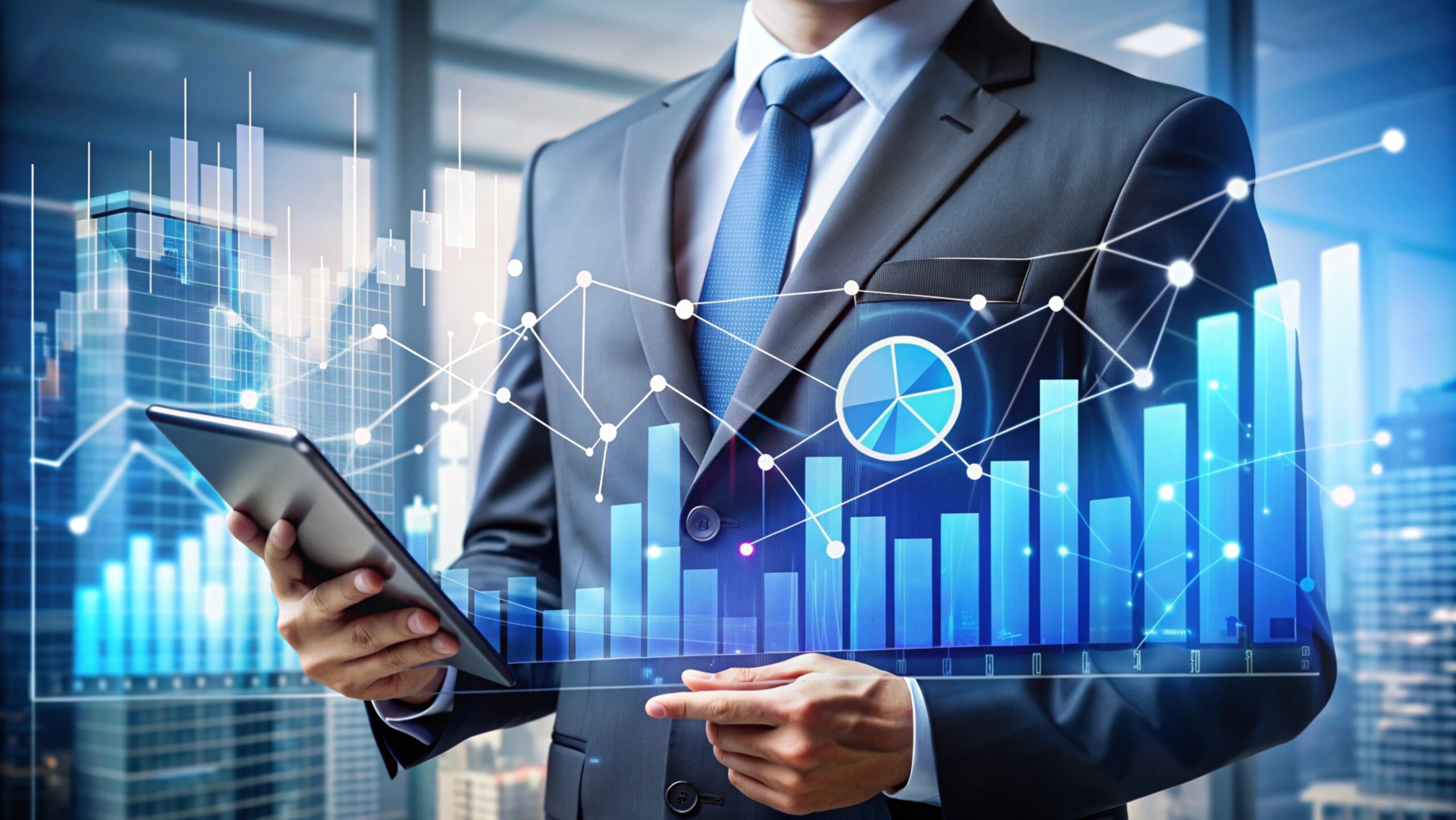
How Do I Handle File Uploads via an API?
Handling file uploads via an API is a crucial aspect of many web and mobile applications. Whether you’re dealing with user-generated content, financial documents, or market data, ensuring a smooth and secure upload process is essential. This guide will walk you through the fundamental steps, best practices, and tools to manage file uploads effectively.
Understanding File Uploads in an API
File uploads typically involve sending a file from a client (web browser, mobile app, or another server) to an API endpoint. The API processes the file and stores it in a database, cloud storage, or another repository.
Common Use Cases for File Uploads
- Uploading financial reports and transaction records.
- Importing large datasets for market analysis.
- Storing user profile pictures and documents.
Setting Up API File Uploads
1. Choose the Right HTTP Method
APIs handle file uploads through HTTP methods such as:
- POST: Used for uploading new files.
- PUT: Used for updating existing files.
2. Define the API Endpoint
A typical API endpoint for file uploads might look like this:
POST /api/upload
3. Use the Correct Content-Type
For file uploads, the multipart/form-data content type is the most common format. It allows sending both file data and additional metadata in the request.
Example request using cURL:
curl -X POST https://api.insightease.com/upload \
-H “Authorization: Bearer YOUR_API_KEY” \
-F “file=@data.csv”
Handling File Uploads Securely
1. Implement Authentication and Authorization
Ensure only authorized users can upload files. Use API keys, OAuth tokens, or JWT for authentication.
2. Validate File Types and Sizes
Restrict uploads to specific file types and set size limits to prevent abuse.
Example:
ALLOWED_EXTENSIONS = {“csv”, “xlsx”, “json”}
MAX_FILE_SIZE = 10 * 1024 * 1024 # 10MB
def validate_file(file):
if file.filename.split(“.”)[-1] not in ALLOWED_EXTENSIONS:
return False, “Unsupported file type”
if len(file.read()) > MAX_FILE_SIZE:
return False, “File size exceeds limit”
return True, “File is valid”
3. Use Secure Storage Solutions
Store files securely using cloud storage services like Amazon S3, Google Cloud Storage, or Azure Blob Storage.
Example of uploading a file to AWS S3:
import boto3
s3 = boto3.client(“s3”)
s3.upload_file(“localfile.csv”, “mybucket”, “uploadedfile.csv”)
4. Encrypt Sensitive Data
Use encryption to protect sensitive files during transmission and storage. TLS (HTTPS) should be enabled for secure communication.
5. Monitor and Log Upload Activity
Track upload attempts and failures using logging mechanisms to detect anomalies.
Handling Large File Uploads
1. Chunked Uploads
For large files, split them into smaller chunks and upload them sequentially.
2. Background Processing
Use asynchronous processing (e.g., message queues) to handle large file uploads without blocking other API requests.
Example with Celery:
from celery import Celery
app = Celery(“tasks”, broker=”redis://localhost:6379″)
@app.task
def process_file(file_path):
# File processing logic
return “Processing complete”
Testing and Debugging File Uploads
Use tools like Postman, cURL, and API clients to test file uploads and handle errors effectively.
Example response for a successful upload:
{
“message”: “File uploaded successfully”,
“file_url”: “https://storage.insightease.com/uploads/data.csv”
}
Conclusion
Implementing secure and efficient file uploads in an API requires careful planning, validation, and monitoring. By following best practices, using secure storage solutions, and handling large files effectively, you can create a reliable file upload system that integrates seamlessly with your API.