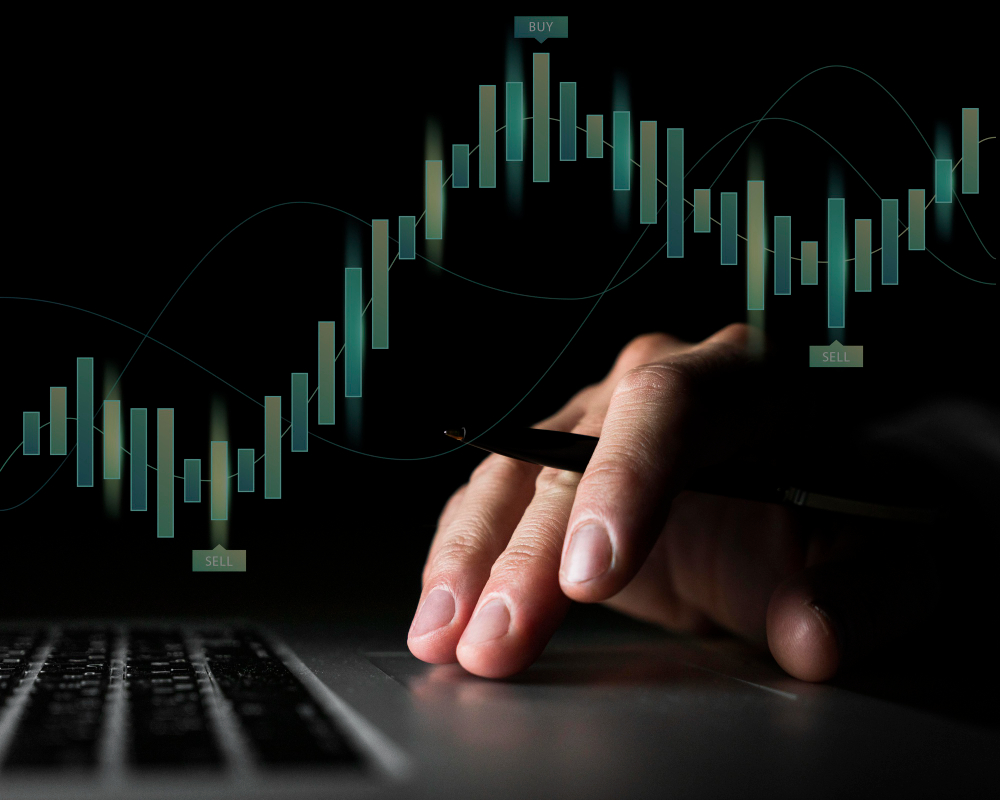
10 Code Snippets for Working with InsightEase Financial APIs
For developers building tools for brokers, investors, traders, and financial professionals, integrating reliable financial data is essential. InsightEase (insightease.com) provides a comprehensive API suite, delivering real-time and historical data for forex, cryptocurrencies, stocks, and commodities. Below are 10 practical code snippets to help you work with InsightEase financial APIs, streamlining integration into trading platforms and applications.
Getting Started with InsightEase APIs
Before using these snippets, sign up at insightease.com to obtain your API key. InsightEase offers seamless integration, live charts, customizable dashboards, market analysis, and an economic calendar—valuable features for financial applications.
Prerequisites
Ensure you have your API key stored securely and a programming environment (e.g., Python, JavaScript) set up. These examples assume basic familiarity with API requests.
Code Snippets for Key Use Cases
1. Fetch Live Stock Prices (Python)
Retrieve real-time stock data, such as Apple’s current price:
python
CollapseWrapCopy
import requests
url = “https://api.insightease.com/v1/stocks/rates?symbol=AAPL”
headers = {“Authorization”: “Bearer YOUR_API_KEY”}
response = requests.get(url, headers=headers)
data = response.json()
print(f”Apple Stock Price: {data[‘rates’][‘AAPL’]}“)
2. Get Crypto Rates (JavaScript)
Pull live Bitcoin prices:
javascript
CollapseWrapCopy
const axios = require(‘axios’);
axios.get(‘https://api.insightease.com/v1/crypto/rates?symbol=BTCUSD’, {
headers: { ‘Authorization’: ‘Bearer YOUR_API_KEY’ }
})
.then(response => console.log(`Bitcoin Price: ${response.data.rates.BTCUSD}`));
3. Stream Forex Data (JavaScript WebSocket)
Stream live EUR/USD rates:
javascript
CollapseWrapCopy
const WebSocket = require(‘ws’);
const ws = new WebSocket(‘wss://api.insightease.com/v1/forex/stream?symbol=EURUSD’, {
headers: { ‘Authorization’: ‘Bearer YOUR_API_KEY’ }
});
ws.on(‘message’, (data) => console.log(`EUR/USD: ${JSON.parse(data).rates.EURUSD}`));
4. Historical Stock Data (Python)
Fetch Apple’s past week data:
python
CollapseWrapCopy
url = “https://api.insightease.com/v1/stocks/history?symbol=AAPL&period=7d”
headers = {“Authorization”: “Bearer YOUR_API_KEY”}
response = requests.get(url, headers=headers)
data = response.json()
print(data[‘history’])
5. Multi-Asset Rates (Python)
Get rates for multiple assets:
python
CollapseWrapCopy
url = “https://api.insightease.com/v1/rates?symbols=AAPL,BTCUSD,EURUSD”
headers = {“Authorization”: “Bearer YOUR_API_KEY”}
response = requests.get(url, headers=headers)
print(response.json()[‘rates’])
6. Economic Calendar Events (JavaScript)
Retrieve upcoming events:
javascript
CollapseWrapCopy
fetch(‘https://api.insightease.com/v1/economic-calendar’, {
headers: { ‘Authorization’: ‘Bearer YOUR_API_KEY’ }
})
.then(response => response.json())
.then(data => console.log(data.events));
7. Crypto Volume Data (Python)
Fetch Bitcoin trading volume:
python
CollapseWrapCopy
url = “https://api.insightease.com/v1/crypto/volume?symbol=BTCUSD”
headers = {“Authorization”: “Bearer YOUR_API_KEY”}
response = requests.get(url, headers=headers)
print(f”Bitcoin Volume: {response.json()[‘volume’]}“)
8. Live Chart Data (JavaScript)
Prepare data for charting:
javascript
CollapseWrapCopy
axios.get(‘https://api.insightease.com/v1/stocks/rates?symbol=TSLA’, {
headers: { ‘Authorization’: ‘Bearer YOUR_API_KEY’ }
})
.then(response => {
const chartData = { label: ‘Tesla’, price: response.data.rates.TSLA };
console.log(chartData);
});
9. Commodities Rates (Python)
Get gold prices:
python
CollapseWrapCopy
url = “https://api.insightease.com/v1/commodities/rates?symbol=XAUUSD”
headers = {“Authorization”: “Bearer YOUR_API_KEY”}
response = requests.get(url, headers=headers)
print(f”Gold Price: {response.json()[‘rates’][‘XAUUSD’]}“)
10. Error Handling (JavaScript)
Handle API errors gracefully:
javascript
CollapseWrapCopy
axios.get(‘https://api.insightease.com/v1/crypto/rates?symbol=ETHUSD’, {
headers: { ‘Authorization’: ‘Bearer YOUR_API_KEY’ }
})
.then(response => console.log(response.data.rates.ETHUSD))
.catch(error => console.error(‘API Error:’, error.response.status));
Why These Snippets Matter
These examples demonstrate how InsightEase APIs support diverse needs—live rates for traders, historical data for investors, and event tracking for brokers. Their flexibility enhances fintech projects with minimal effort.
Tailoring to Your Project
Adjust parameters like symbols or timeframes to fit your app’s requirements, leveraging InsightEase’s broad market coverage.
Conclusion
InsightEase (insightease.com) equips developers with powerful financial APIs to create tools for brokers, investors, traders, and financial professionals. These 10 code snippets showcase how to harness real-time stock, crypto, forex, and commodities data, alongside features like live charts and market analysis. Visit insightease.com to explore their services and start building robust financial applications today.